A TypeScript-like language for WebAssembly.
Designed for WebAssembly
AssemblyScript targets WebAssembly's feature set specifically, giving developers low-level control over their code.
Familiar TypeScript syntax
Its similarity with TypeScript makes it easy to compile to WebAssembly without learning a new language.
Right at your fingertips
Integrates with the existing Web ecosystem - no heavy toolchains to set up. Simply npm install
it!
#!runtime=stub
/** Calculates the n-th Fibonacci number. */
export function fib(n: i32): i32 {
var a = 0, b = 1
if (n > 0) {
while (--n) {
let t = a + b
a = b
b = t
}
return b
}
return a
}
#!html
<textarea id="output" style="height: 100%; width: 100%" readonly></textarea>
<script type="module">
const exports = await instantiate(await compile(), { /* imports */ })
const output = document.getElementById('output')
for (let i = 0; i <= 10; ++i) {
output.value += `fib(${i}) = ${exports.fib(i)}\n`
}
</script>
AssemblyScript is free and open source software released under the Apache License, Version 2.0, builds upon Binaryen and is based on the WebAssembly specification. It is brought to you by the following awesome people:
Why AssemblyScript?
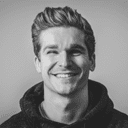
“You are now able to write WebAssembly without learning a new language, and harness all these benefits WebAssembly might offer you. I think that is kind of powerful. AssemblyScript is absolutely usable, and very enjoyable!” – Surma, WebAssembly for Web Developers (Google I/O ’19)
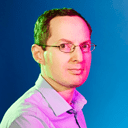
“AssemblyScript is designed with WebAssembly and code size in mind. It's not an existing language that we are using for a new purpose but it's a language designed for WebAssembly. It has great wasm-opt integration, in fact it's built with it, and it's very easy to get good code size.” – Alon Zakai, Shipping Tiny WebAssembly Builds (WebAssembly Summit)
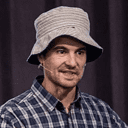
“I chose AssemblyScript because it has high-level readability and low-level control. It's like a high-level language but you get that low-level feeling and you can even directly write WebAssembly intrinsics if you want to.” – Peter Salomonsen, WebAssembly Music (WebAssembly Summit)
Thanks to our sponsors!
Most of the maintainers and contributors do this open source work in their free time. If you use AssemblyScript for a serious task or plan to do so, and you'd like us to invest more time on it, please donate to our OpenCollective. By sponsoring this project, your logo will show up below. Thank you so much for your support!
Join our Discord
If you have questions only a human can answer, would like to show others what you are working on or just want to hang out with other AssemblyScript folks, make sure to join our Discord server! There you'll find channels for #announcements, #help, and more.